How To Use ChatGPT API In Python
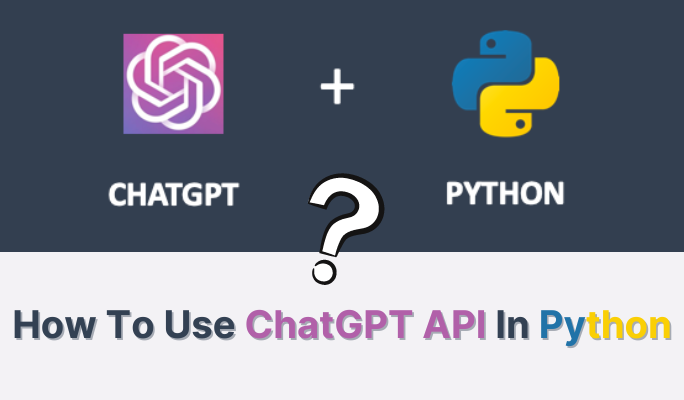
ChatGPT API Python
ChatGPT, as you may know, is a popular language model created by OpenAI with its ability to have conversations that sound just like a human.
OpenAI has a website, https://chat.openai.com, where you can chat with ChatGPT. They have also created an API that lets developers add ChatGPT to their own applications. In this tutorial, you'll learn how to use the ChatGPT API with Python.
Requirements
Before we begin, ensure that you have the following things prepared:
- Python 3.7 or a newer version. If your computer doesn't have Python, don't worry. You can visit python.org and get it by downloading from that website.
- An OpenAI API key. You can request access to it by clicking on the link provided.
To begin, we have to set up Python on your computer. This will allow you to write and run Python programs easily. After that, we'll add ChatGPT's API to your Python applications. It's just like adding any other Python library to your project.
To use ChatGPT and generate text, you'll need to create an API key and install OpenAI's client. Once you’ve created your new secret key, here’s how to use ChatGPT with Python.
How To Use ChatGPT With Python
The most popular way to use ChatGPT in Python is to build a chatbot. To create a chatbot using the ChatGPT API and Python, you'll start by writing a function that communicates with the ChatGPT API. In this example, we'll use the model GPT-3.5 turbo, which is great for chatbots.
Here's a simple function that sends messages to the ChatGPT API and receives a response:
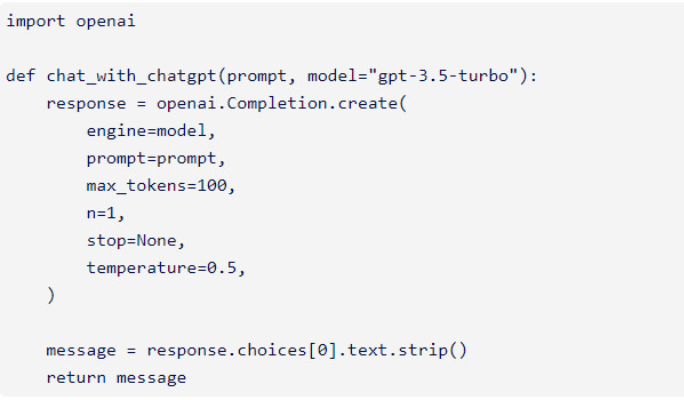
ChatGPT API Python Response 1
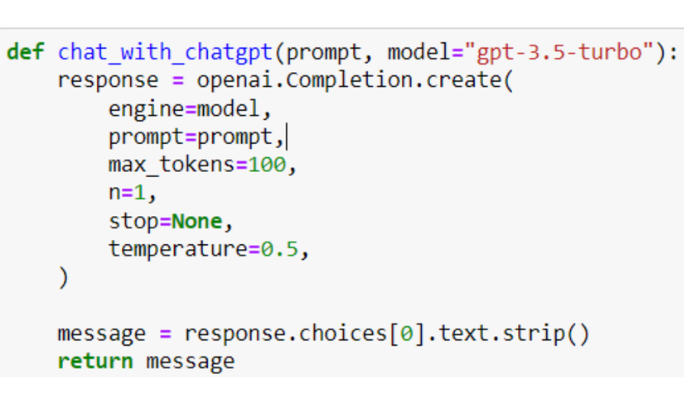
ChatGPT API Python Response 2
The Python code above includes the OpenAI library and a function called 'chat_with_chatgpt.' This function is responsible for generating responses using the ChatGPT model through the OpenAI API. It takes a user prompt as input and returns the generated response as a text message.
To use this function, you can call it with user input. The following command will give you the generated response:
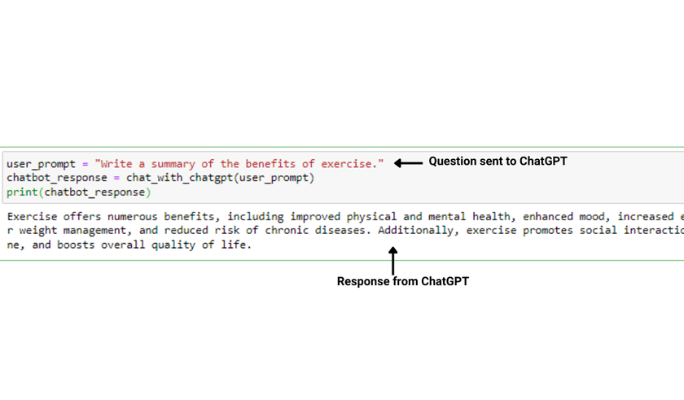
ChatGPT API Python Response 3
With this setup, you are successfully integrating ChatGPT into your Python application. This allows you to create various types of text-based interactions using the powerful ChatGPT model.
You can customize the parameters of the function according to your project requirements. If you want to know more about how to use the API and what different things you can do with it, you can check the developer documentation. It has all the detailed information you need to learn and explore.
Next, we will explore how to use OpenAI ChatGPT's messaging prompt feature for writing Python code.
4 Python Code Examples To Employ ChatGPT
ChatGPT has revolutionized the way developers write code, allowing them to improve their code quality and save time in the process. With the messaging prompt feature of ChatGPT, developers can write code more efficiently.
In this section, we will explore four examples that will help you understand how to leverage ChatGPT for writing complex code. These examples will demonstrate how ChatGPT can enhance your productivity when developing applications or working with data in Python.
By the end of this section, you will have a solid understanding of how to harness the power of ChatGPT to write better and faster code. Let's dive in.
Example 1: Asking ChatGPT To Create Tricky Algorithm
Algorithm is like a set of instructions that a computer can follow to do something. Sometimes, writing these instructions can take a long time.
But with ChatGPT, things have become easier. You can ask ChatGPT to write complex instructions for you in a language called Python. It's as simple as asking ChatGPT to do it.
Let's try an example. We'll ask ChatGPT to write instructions for finding something called the longest common subsequence between two groups of letters. This helps us find the similarities between the groups.
The longest common subsequence idea is used in many fields. For example, in bioinformatics, scientists compare DNA, RNA, or protein sequences using this idea. It's also used in text processing to find similarities between words or to see how different two strings are.

ChatGPT API Python Example 1
You can take the code and use it in your Python project. You can make changes to the code so that it does what you want it to do.
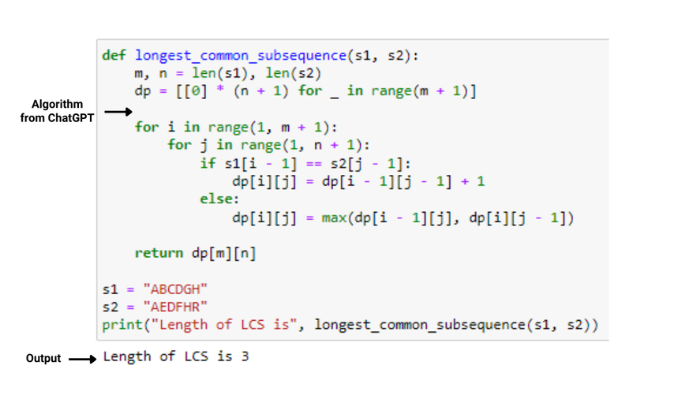
ChatGPT API Python Example 2
By asking ChatGPT to write this set of instructions for us, we can save time and easily use this cool feature in our Python programs.
Example 2: Asking ChatGPT To Help With Getting Information From Websites
Have you ever wanted to get information from a website but thought it was too difficult? Well, ChatGPT is here to help. It can make it easier and faster for you to write code that can get information from websites.
For example, we'll use something called the BeautifulSoup library to extract all the headings from a webpage. This means we can get the main titles or headings from a website without any trouble:

ChatGPT API Python Example 3
When you use the code in your Python project, you only need to change the website address (URL) to the one you want to get information from. If you want to scrape something different from website headings, you can ask ChatGPT to help you modify the code.
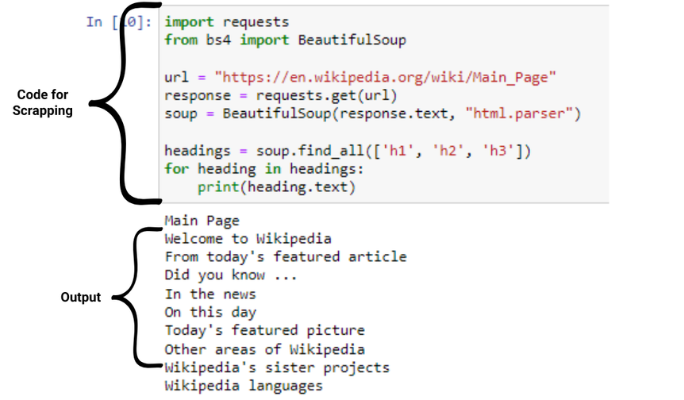
ChatGPT API Python Example 4
It's like having a helpful friend who can make the code work for different things you want to scrape.
Example 3: Asking ChatGPT To Help Analyze Data Using Python
You can use ChatGPT to help you with data analysis too. In the next program we'll show you, ChatGPT helps us do some cool things with a bunch of numbers. We can import the data, filter it, and even find the average (mean) of the numbers.

ChatGPT API Python Example 5
Once you finish writing the code, you can copy it to your Python project. If you want to make the code do something different to fit your needs, you can make changes to it as well.
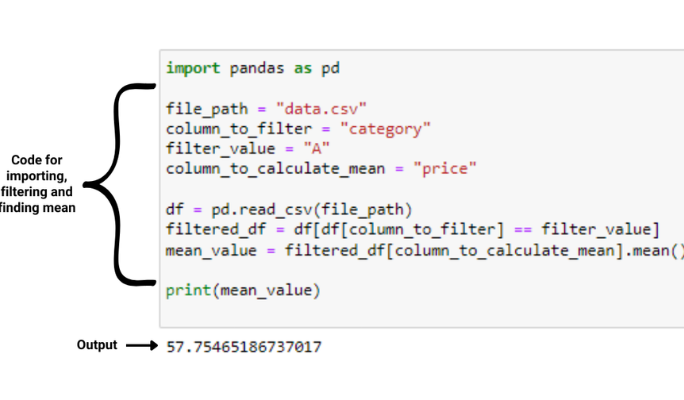
ChatGPT API Python Example 6
With ChatGPT, you can create advanced machine learning models. These models, like Decision Trees and Logistic Regressions, can make complex decisions and predictions. You can use these models in your Python code to enhance your programs and solve more advanced problems.
Example 4: Asking ChatGPT To Make Web Apps With Flask
With ChatGPT, you can write code to make your own web applications using Python. Let's look at an example. We can create a simple web app that says "Hello, World!" when you visit it:

ChatGPT API Python Example 7
This example is a great way for beginners to start learning about web development or using Flask. It shows how to create a basic part of a website, called a route, and a special function that goes with it. You can use this as a starting point and add more routes and features to make your website even more exciting.
All the examples we've seen show how amazing ChatGPT is at helping us generate snippets of code in Python for different tasks. By using ChatGPT, developers can get quick help in solving difficult problems or creating simple functions.
Frequently Asked Questions
ChatGPT is like a ready-to-use program that you can use directly in your web browser. On the other hand, ChatGPT API is like a special tool that programmers can use in their own code. They can get it and use it with different programming languages to make their own cool programs.
Currently, there is no free version of ChatGPT API available. However, OpenAI provides a free trial period for new users to try out the API before deciding if they want to pay for it. During the trial period, you can try it out and see how it works. If you decide to keep using it after the trial, there will be a cost to use the API.
Based on our experience, ChatGPT is often helpful, but it's important to remember that nothing can replace learning and gaining real-world experience.
It's always a good idea to double-check your work and use ChatGPT as a tool to enhance what you're doing, rather than relying on it completely. It's possible that things might change in the future, so it's essential to keep learning and growing your own knowledge and skills.
No, ChatGPT will not completely replace programmers. However, it can help with certain parts of programming by automatically generating code, fixing errors, and creating documentation.
ChatGPT has the ability to learn from lots of code and information, so it can generate new code that is similar to existing examples. While it can be a useful tool, programmers still play a crucial role in designing, planning, and solving complex problems in software development.
ChatGPT is based on the GPT-3.5 architecture, which uses a special deep learning algorithm called a transformer. This algorithm is designed to understand and generate human-like language.
It uses a big model that has learned from lots and lots of text data. So when you ask ChatGPT a question, it uses this algorithm to come up with a response that sounds like something a human would say.
Conclusion
In this article, we've talked about how ChatGPT can help us with Python programming. It can be used in different ways, like adding it to our own programs or getting help with our code using messages.
By using ChatGPT, we can work faster, make more complicated programs, and learn more about Python. But it's important to remember that we should still learn by ourselves and not rely only on ChatGPT.
The examples we've seen in this article show how ChatGPT can help us write better code quickly. By using ChatGPT alongside our own learning, we can elevate our programming skills to new heights